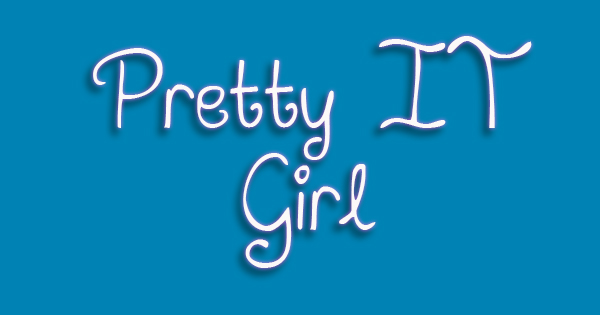
Check out the codes below:
DISMISSING THE KEYPAD:
- (void)dismissKeyboard { [self.view endEditing:YES]; }
UITextField Delegates (Oh, I forgot to mention that you need to set the delegate of your UITextFields. Set the delegates to self, also put the UITextField Class delegate to your .h file.
- (void)textFieldDidBeginEditing:(UITextField *)textField { [self animateTextField: textField up: YES]; } - (void)textFieldDidEndEditing:(UITextField *)textField { [self animateTextField: textField up: NO]; }
Now, for the ANIMATION part:
- (void)animateTextField: (UITextField*) textField up: (BOOL) up { const int movementDistance = 80; // tweak as needed const float movementDuration = 0.3f; // tweak as needed int movement = (up ? -movementDistance : movementDistance); [UIView beginAnimations: @"anim" context: nil]; [UIView setAnimationBeginsFromCurrentState: YES]; [UIView setAnimationDuration: movementDuration]; self.view.frame = CGRectOffset(self.view.frame, 0, movement); [UIView commitAnimations]; }
If the tutorial is not clear enough for you, please feel free to shoot me a question or your questions by commenting below of the post or contacting me.
Post a Comment