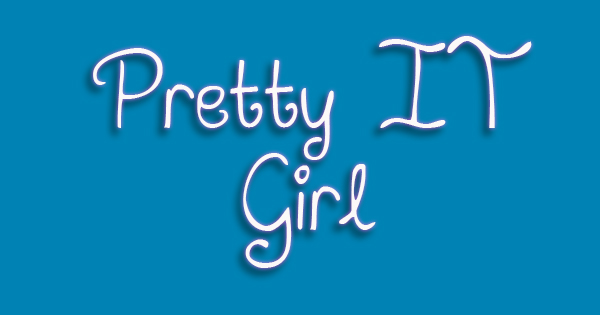
I wanna show you today how I masked my image for my MKMapView in my project. Basically, masking is a method of laying out your IMAGE 1 to the top of the IMAGE 2, and that IMAGE 2 will act as your pattern and the product will be the IMAGE 1 taking out the shape of the IMAGE 2. So if you wanna draw something in iOS programmatically, without using complex ways, just draw an image you want in Photoshop or in Paintbrush and use that image as your pattern to draw what you want.
Here it goes: (by the way, I got this from a certain question on SO - but this answer is not really selected as an answer)
(UIImage*) maskImage:(UIImage *)image withMask:(UIImage *)maskImage { CGColorSpaceRef colorSpace = CGColorSpaceCreateDeviceRGB(); CGImageRef maskImageRef = [maskImage CGImage]; // create a bitmap graphics context the size of the image CGContextRef mainViewContentContext = CGBitmapContextCreate (NULL, maskImage.size.width, maskImage.size.height, 8, 0, colorSpace, kCGImageAlphaPremultipliedLast); CGColorSpaceRelease(colorSpace); if (mainViewContentContext==NULL) return NULL; CGFloat ratio = 0; ratio = maskImage.size.width/ image.size.width; if(ratio * image.size.height < maskImage.size.height) { ratio = maskImage.size.height/ image.size.height; } CGRect rect1 = {{0, 0}, {maskImage.size.width, maskImage.size.height}}; CGRect rect2 = {{-((image.size.width*ratio)-maskImage.size.width)/2 , -((image.size.height*ratio)-maskImage.size.height)/2}, {image.size.width*ratio, image.size.height*ratio}}; CGContextClipToMask(mainViewContentContext, rect1, maskImageRef); CGContextDrawImage(mainViewContentContext, rect2, image.CGImage); // Create CGImageRef of the main view bitmap content, and then // release that bitmap context CGImageRef newImage = CGBitmapContextCreateImage(mainViewContentContext); CGContextRelease(mainViewContentContext); UIImage *theImage = [UIImage imageWithCGImage:newImage]; CGImageRelease(newImage); // return the image return theImage; }
See? No need to explain the method above furthermore. You just pass your UIImages to that method - with parameter image acting as your square image (usually square - the normal shape) or whatever image you want to change its shape into a certain form. Then the maskImage is the IMAGE 2 that I was talking about, this will act as your pattern and your IMAGE 1 will take the shape of that IMAGE 2.
If this code helped you, say thanks by sharing this post and commenting out your suggestiions. Thank you!
Post a Comment